Collection Hierarchy
After knowing the meaning and purpose of Collections framework, let us know the basic interfaces, derived classes and their properties. Java data structures are nothing but subclasses of Collection and Map interfaces. Following hierarchy gives all the interfaces of Collections framework.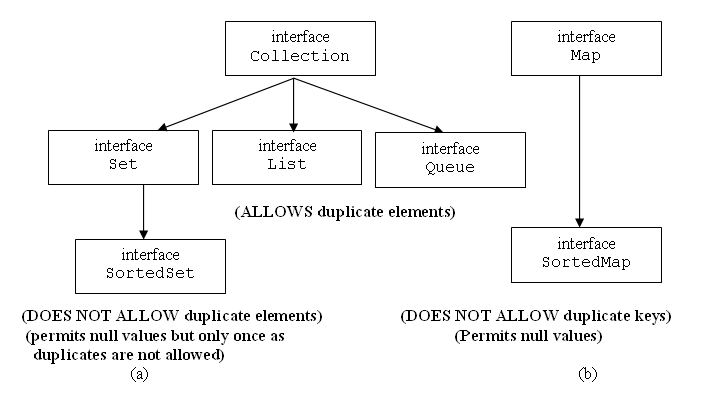
Note: Framework name is collections and the interface name is Collection. Do not get confused; later, we get one more class called Collections.
Description of fundamental interfaces
A table is given showing the core interfaces with their implemented classes with brief description.
Interface | Brief Description | Derived Classes |
---|---|---|
Collection | It forms the root interface and comes with methods that define basic operations and makes all DS as one unit (called by a common name) | Majority of all DS come under this interface |
Set | Subclasses of this interface have a common feature of not allowing duplicate elements | HashSet, LinkedHashSet |
SortedSet | Subclasses of this interface come with a common feature of printing the elements in ascending order implicitly. Earlier feature derived from Set is allowing only unique elements | TreeSet |
List | Subclasses allow duplicate elements | LinkedList, ArrayList, Vector |
Map | Allows the subclasses to store key/value pairs. Does not allow duplicate keys. | HashMap, LinkedHashMap, Hashtable |
SortedMap | Prints elements in ascending order of keys. A feature derived from Map is it does not allow duplicate keys. | TreeMap |
The elements of List can be accessed with their index numbers.
Collection classes store elements of single entities, but Map stores key/value pairs; to store a value, a key must be supplied along. SortedSet and SortedMap print their stored elements in ascending order (known as natural order) by default. The order can be customized explicitly using Comparator interface.
Following figure displays the main interfaces with relation to their derived classes.

As it can be observed, there comes some abstract classes in between interfaces and concrete classes (DS classes). They add some extra features to subclasses other than inherited from interfaces.
Following table gives the properties of derived classes.
Data Structure | Interface | Duplicates | Methods available | DS that inherits |
---|---|---|---|---|
HashSet | Set | Unique elements | equals(), hashCode() | Hashtable |
LinkedHashSet | Set | Unique elements | equals(), hashCode() | Hashtable, doubly-linked list |
TreeSet | SortedSet | Unique elements | equals(), compareTo() | Balanced Tree |
ArrayList | List | Duplicates allowed | equals() | Resizable array |
LinkedList | List | Duplicate allowed | equals() | Linked list |
Vector | List | Duplicates allowed | equals() | Resizable array |
HashMap | Map | Unique keys | equals() and hashCode() | Hash table |
LinkedHashMap | Map | Unique keys | equals() and hashCode() | Hash table and doubly-linked list |
Hashtable | Map | Unique keys | equals(), hashCode() | Hash table |
TreeMap | SortedMap | Unique keys | equals(), compareTo() | Tree Map |
There are two distinct hierarchies monitored by Collection and Map. Map is also placed in collections framework to have interoperability.
Basic Features of Main Interfaces
- Collection is the root interface for all the hierarchy (except Map).
- Set interface unique feature is that it does not accept duplicate elements. That is, no two elements will be the same.
- SortedSet interface is derived from Set interface and adds one more feature that the elements are arranged in sorted order by default.
- List interface permits duplicate elements.
- Queue interface holds elements and returns in FIFO order.
- Map adds the elements in key/value pairs. Duplicate keys are not allowed, but duplicate values are allowed. One key can map one value only.
- SortedMap interface is a particular case of Map. Keys are sorted by default.